En este tutorial aprenderemos a activar, gestionar y probar Bluetooth en un ESP32 utilizando el lenguaje de programación Arduino. Bluetooth es una tecnología inalámbrica ampliamente utilizada para la comunicación entre dispositivos electrónicos. Puedes convertir rápidamente tu sistema en un objeto conectado.
Equipamiento
- Un módulo ESP32 (Bluetooth+Wifi a bordo)
- Un ordenador con Python instalado o un smartphone
- Cable USB para conexión ESP32-ordenador
Configuración del entorno y del IDE
Para programar tu ESP32 con el IDE de Arduino, puedes seguir este tutorial anterior.
Recuperar dirección MAC
Esta información no es necesariamente necesaria, pero siempre es una buena idea saber cómo recuperar la dirección MAC del ESP32.
#include "esp_bt_main.h" #include "esp_bt_device.h" #include "BluetoothSerial.h" BluetoothSerial SerialBT; void printDeviceAddress() { const uint8_t* point = esp_bt_dev_get_address(); for (int i = 0; i < 6; i++) { char str[3]; sprintf(str, "%02X", (int)point[i]); Serial.print(str); if (i < 5){ Serial.print(":"); } } } void setup() { Serial.begin(115200); SerialBT.begin("ESP32BT"); Serial.print("MaxAddr : "); printDeviceAddress(); } void loop() {}
Salida
14:42:43.448 -> MaxAddr : 3C:61:05:31:5F:12
Comunicación serie por Bluetooth
La comunicación Bluetooth se activa del mismo modo que la comunicación serie. El método es similar para el módulo HC-06
#include "BluetoothSerial.h" #if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED) #error Bluetooth is not enabled! Please run `make menuconfig` to and enable it #endif BluetoothSerial SerialBT; void setup() { Serial.begin(115200); SerialBT.begin("ESP32BT"); //Bluetooth device name Serial.println("The device started, now you can pair it with bluetooth!"); } void loop() { if (Serial.available()) { SerialBT.write(Serial.read()); } if (SerialBT.available()) { Serial.write(SerialBT.read()); } delay(20); }
N.B.: Parece que hay una forma de añadir un código PIN, pero no consigo que funcione.
SerialBT.setPin(pin);SerialBT.begin("ESP32BT ", true);
Emparejamiento
Una vez configurado el módulo como desee, puede emparejar el ESP32 con el sistema de su elección como cualquier otro dispositivo Bluetooth. Seleccione el nombre de la lista de dispositivos detectados (nombre ESP32BT)
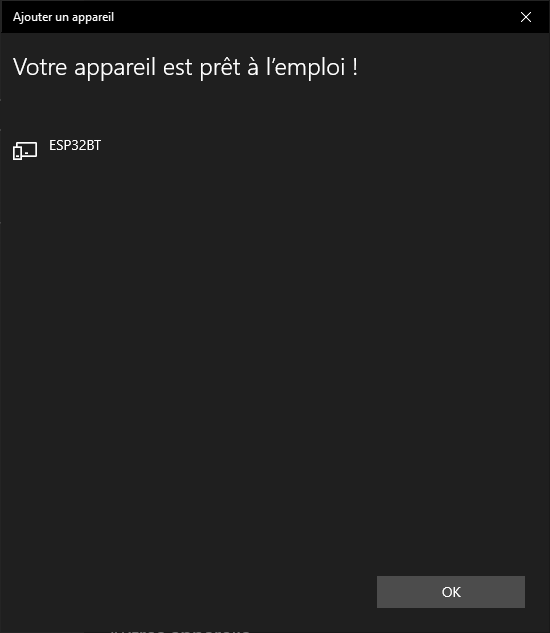
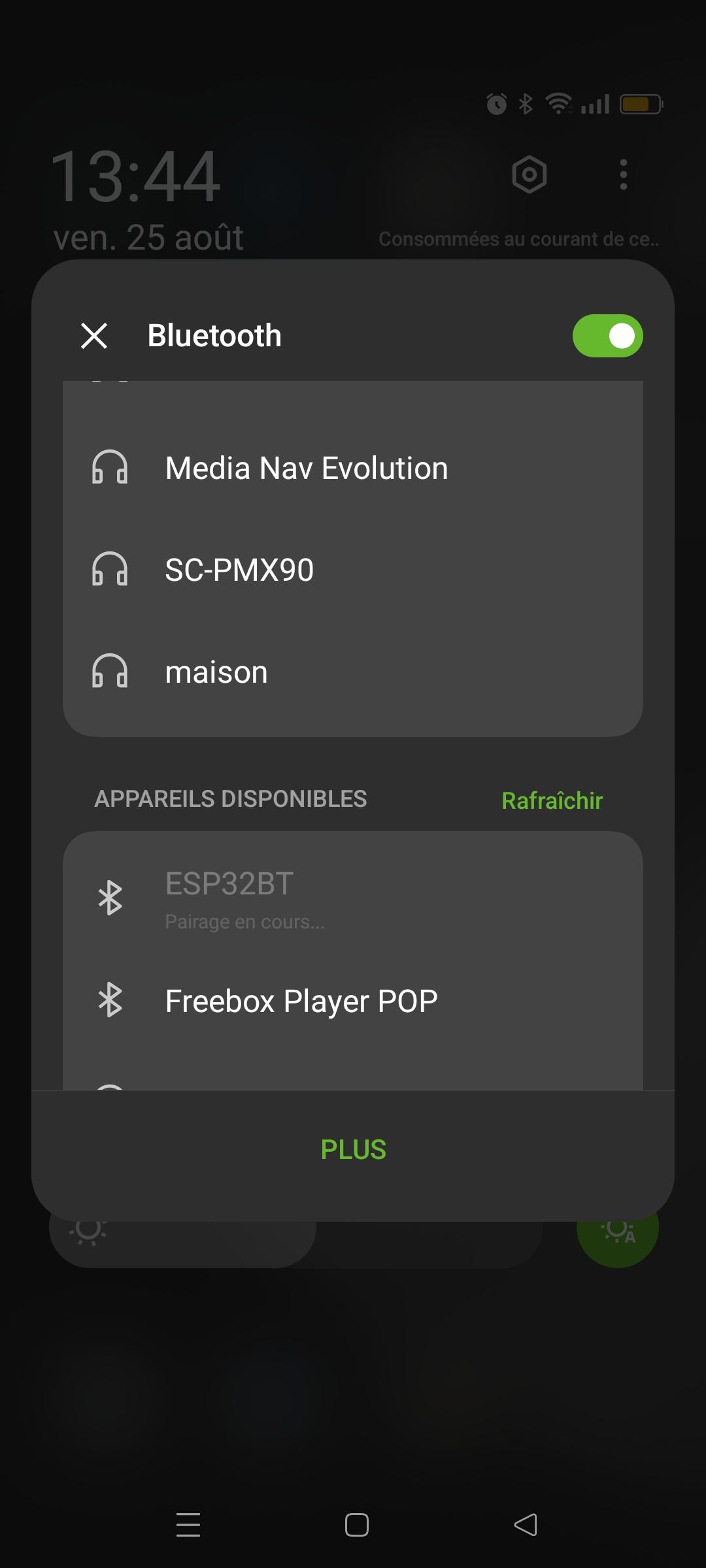
Comprobación de la comunicación Bluetooth mediante un terminal Bluetooth serie
Vamos a probar la comunicación Bluetooth utilizando la aplicación Serial Bluetooth Terminal.
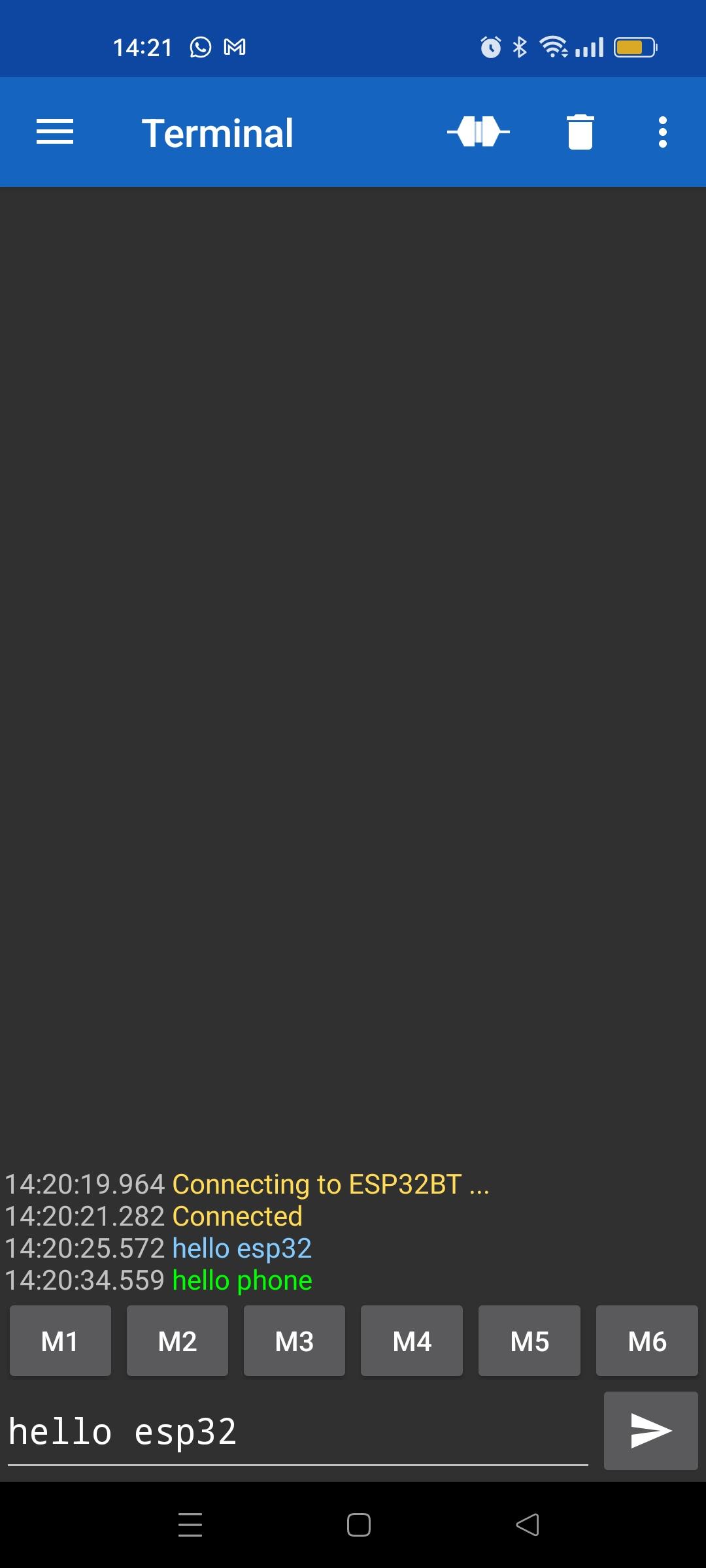
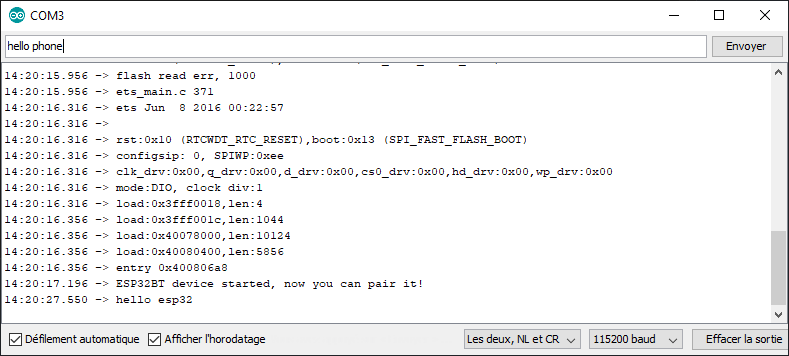
El mensaje se intercambia entre el teléfono y el ESP32 a través de Bluetooth
Código para recuperar el pedido completo
En el código anterior, copiamos el mensaje byte a byte para enviarlo al monitor. Aquí vamos a registrar el comando completo en un String msg. Esto nos permitirá analizar el comando y definir la acción correspondiente (por ejemplo, encender
#include "BluetoothSerial.h" #if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED) #error Bluetooth is not enabled! Please run `make menuconfig` to and enable it #endif String msg; BluetoothSerial SerialBT; const char *pin = "1234"; void setup() { Serial.begin(115200); SerialBT.begin("ESP32BT"); //Bluetooth device name Serial.println("ESP32BT device started, now you can pair it!"); } void loop(){ readSerialPort(); // Send answer to master if(msg!=""){ Serial.print("Master sent : " ); Serial.println(msg); SerialBT.print("received : "+msg); msg=""; } } void readSerialPort(){ while (SerialBT.available()) { delay(10); if (SerialBT.available() >0) { char c = SerialBT.read(); //gets one byte from serial buffer msg += c; //makes the string readString } } SerialBT.flush(); }
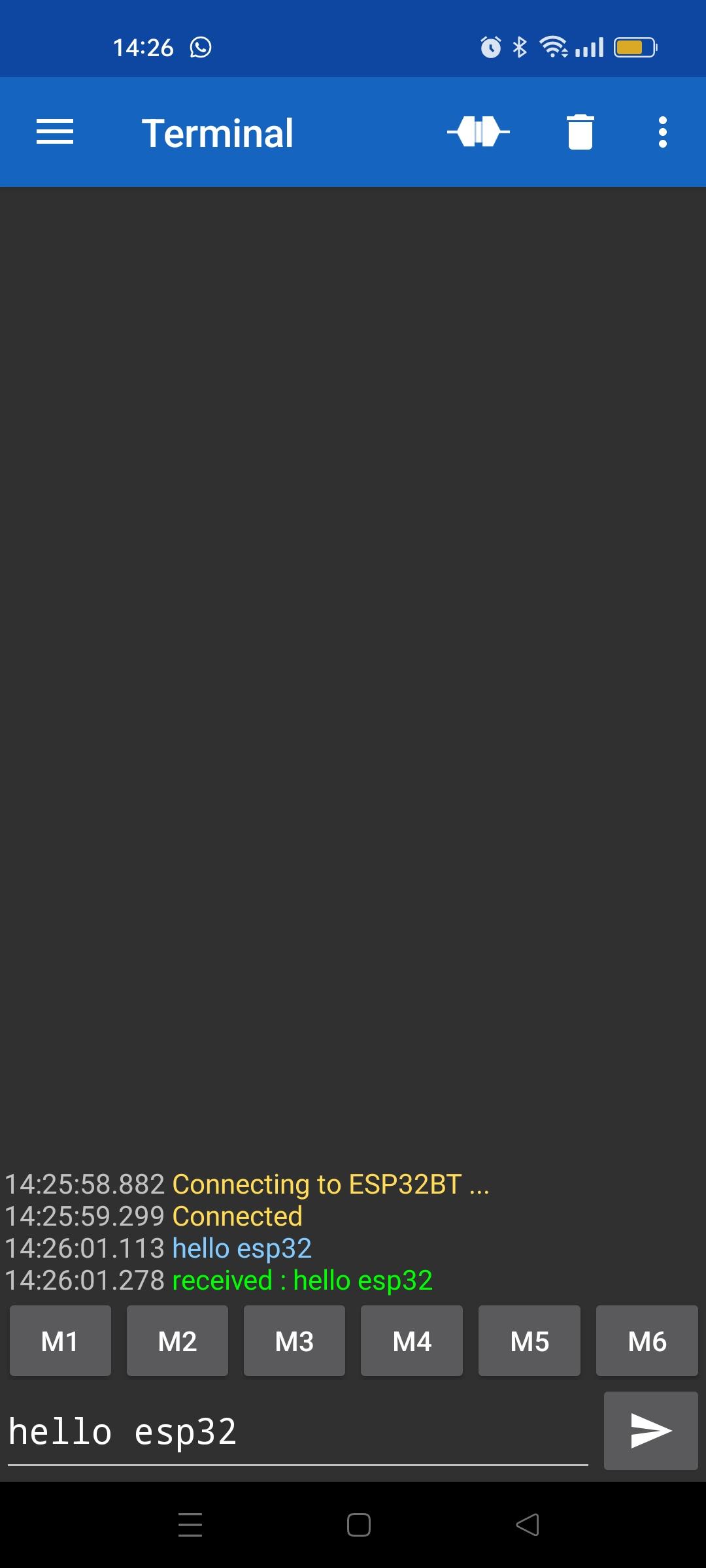
Comunicación entre ESP32 y Python a través de Bluetooth
Puedes gestionar la comunicación Bluetooth desde tu PC.
Para ello, instale el paquete PyBluez
python -m pip install pybluez
Detectar dispositivos bluetooth
import bluetooth target_name = "ESP32BT" target_address = None nearby_devices = bluetooth.discover_devices(lookup_names=True,lookup_class=True) print(nearby_devices) for btaddr, btname, btclass in nearby_devices: if target_name == btname: target_address = btaddr break if target_address is not None: print("found target {} bluetooth device with address {} ".format(target_name,target_address)) else: print("could not find target bluetooth device nearby")
Salida
[('88:C6:26:91:30:84', 'UE\xa0BOOM\xa02', 2360344), ('88:C6:26:7E:F2:7A', 'UE\xa0BOOM\xa02', 2360344), ('4C:EA:AE:D6:92:08', 'OPPO A94 5G', 5898764), ('41:42:DD:1F:45:69', 'MX_light', 2360344), ('3C:61:05:31:5F:12', 'ESP32BT', 7936)] found target ESP32BT bluetooth device with address 3C:61:05:31:5F:12
Conexión y comunicación con el ESP32
He aquí un script en Python para conectarse automáticamente al dispositivo Bluetooth ESP32 desde un PC
import bluetooth import socket target_name = "ESP32BT" target_address = None nearby_devices = bluetooth.discover_devices(lookup_names=True,lookup_class=True) print(nearby_devices) for btaddr, btname, btclass in nearby_devices: if target_name == btname: target_address = btaddr break if target_address is not None: print("found target {} bluetooth device with address {} ".format(target_name,target_address)) """ # With PyBluez NOT WORKING serverMACAddress = target_address port = 1 s = bluetooth.BluetoothSocket(bluetooth.RFCOMM) s.connect((serverMACAddress, port)) while 1: text = raw_input() # Note change to the old (Python 2) raw_input if text == "quit": break s.send(text) data = s.recv(1024) if data: print(data) sock.close()""" serverMACAddress = target_address port = 1 s = socket.socket(socket.AF_BLUETOOTH, socket.SOCK_STREAM, socket.BTPROTO_RFCOMM) s.connect((serverMACAddress,port)) print("connected to {}".format(target_name)) while 1: text = input() if text == "quit": break s.send(bytes(text, 'UTF-8')) data = s.recv(1024) if data: print(data) s.close() else: print("could not find target bluetooth device nearby")
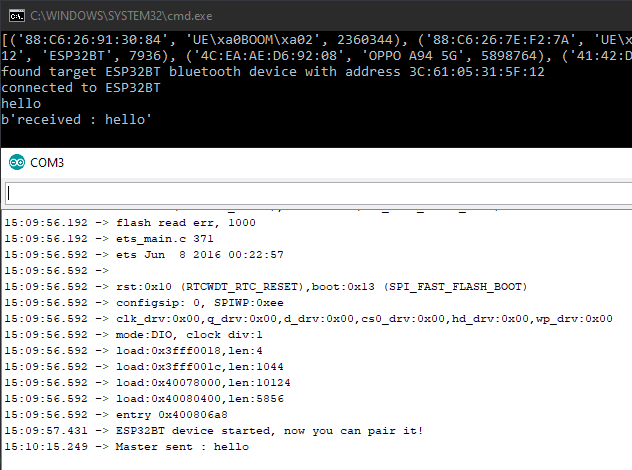
N.B.: Sólo la librería socket funciona para la comunicación Bluetooth. Parece que hay un problema de mantenimiento con la biblioteca PyBluez.