In this tutorial, we’ll learn how to activate, manage and test Bluetooth on an ESP32 using the Arduino programming language. Bluetooth is a wireless technology widely used for communication between electronic devices. It enables you to quickly transform your system into a connected object.
Equipment
- ESP32 module (on-board Bluetooth+Wifi)
- A computer with Python installed or smartphone
- USB cable for ESP32-computer connection
Environment and IDE configuration
To program your ESP32 with the Arduino IDE, you can follow this previous tutorial.
Recover MAC address
This information is not necessarily necessary, but it’s always a good idea to know how to retrieve the MAC address from the ESP32.
#include "esp_bt_main.h" #include "esp_bt_device.h" #include "BluetoothSerial.h" BluetoothSerial SerialBT; void printDeviceAddress() { const uint8_t* point = esp_bt_dev_get_address(); for (int i = 0; i < 6; i++) { char str[3]; sprintf(str, "%02X", (int)point[i]); Serial.print(str); if (i < 5){ Serial.print(":"); } } } void setup() { Serial.begin(115200); SerialBT.begin("ESP32BT"); Serial.print("MaxAddr : "); printDeviceAddress(); } void loop() {}
Output
14:42:43.448 -> MaxAddr : 3C:61:05:31:5F:12
Serial communication via Bluetooth
Bluetooth communication is activated in the same way as serial communication. The method is similar for the HC-06 module
#include "BluetoothSerial.h" #if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED) #error Bluetooth is not enabled! Please run `make menuconfig` to and enable it #endif BluetoothSerial SerialBT; void setup() { Serial.begin(115200); SerialBT.begin("ESP32BT"); //Bluetooth device name Serial.println("The device started, now you can pair it with bluetooth!"); } void loop() { if (Serial.available()) { SerialBT.write(Serial.read()); } if (SerialBT.available()) { Serial.write(SerialBT.read()); } delay(20); }
N.B.: There seems to be a way of adding a PIN code, but I can’t get it to work.
SerialBT.setPin(pin);SerialBT.begin("ESP32BT ", true);
Pairing
Once you’ve configured the module as you wish, you can pair the ESP32 with the system of your choice, just like any other Bluetooth device. Select the name from the list of detected devices (name ESP32BT)
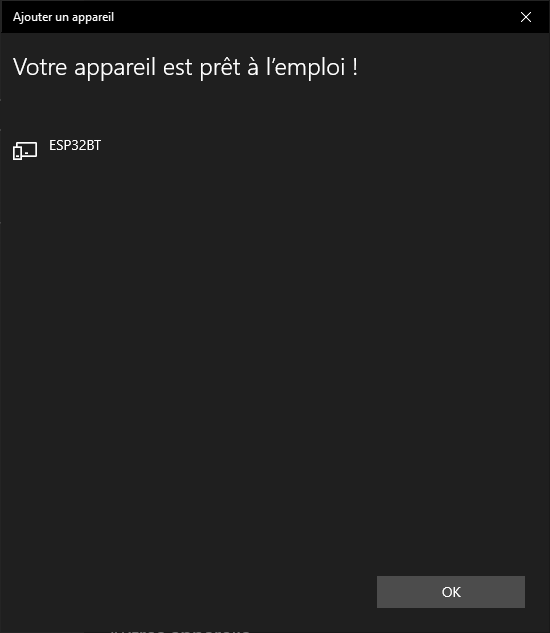
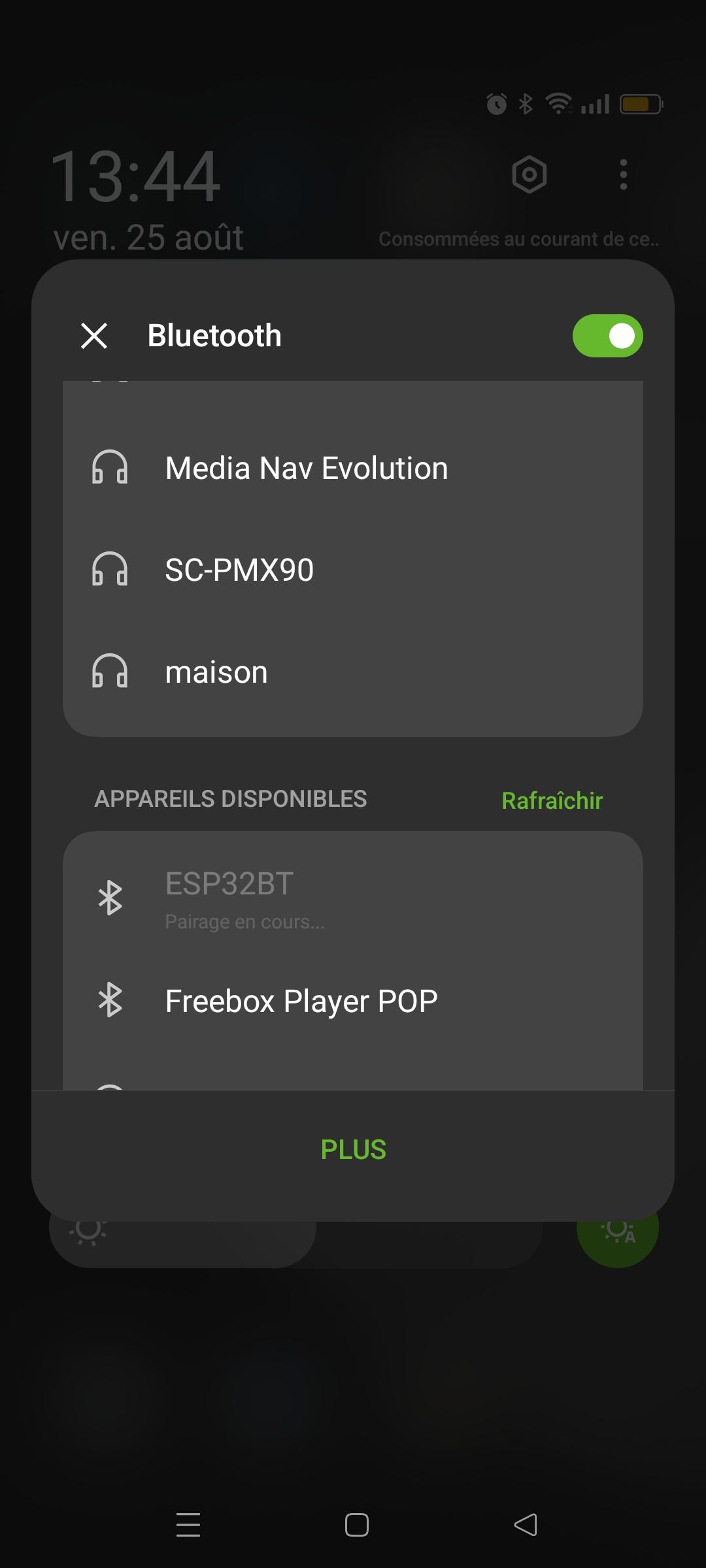
Test Bluetooth communication with Serial Bluetooth Terminal
We’re going to test Bluetooth communication using the Serial Bluetooth Terminal application.
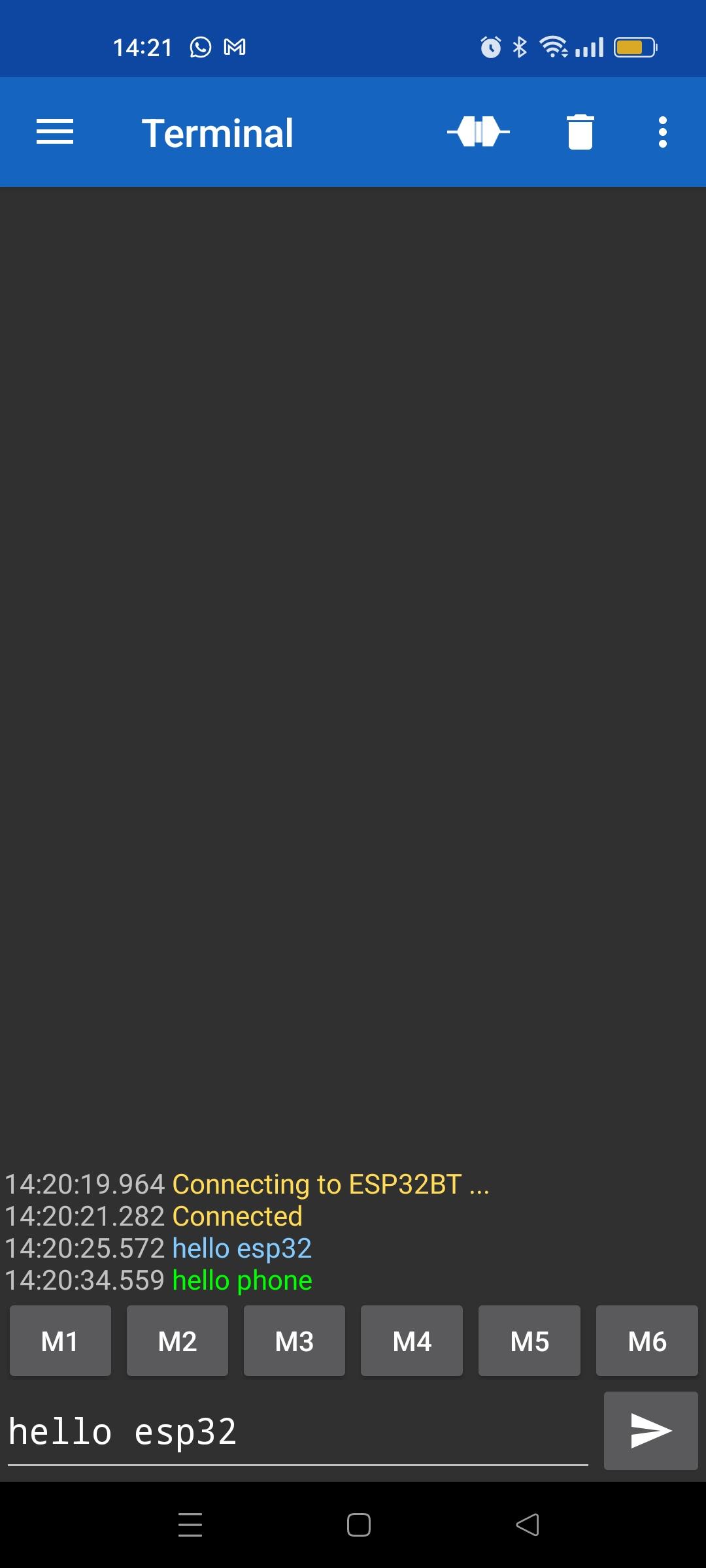
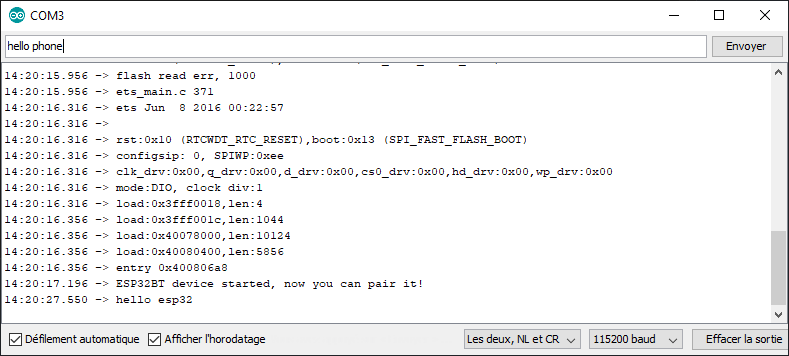
The message is exchanged between the phone and the ESP32 via Bluetooth
Code to retrieve complete order
In the previous code, we made a byte-by-byte copy of the message to send it back to the monitor. Here, we’ll save the complete command in a String msg. This will enable you to analyze the command and define the corresponding action (e.g.: switch on
#include "BluetoothSerial.h" #if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED) #error Bluetooth is not enabled! Please run `make menuconfig` to and enable it #endif String msg; BluetoothSerial SerialBT; const char *pin = "1234"; void setup() { Serial.begin(115200); SerialBT.begin("ESP32BT"); //Bluetooth device name Serial.println("ESP32BT device started, now you can pair it!"); } void loop(){ readSerialPort(); // Send answer to master if(msg!=""){ Serial.print("Master sent : " ); Serial.println(msg); SerialBT.print("received : "+msg); msg=""; } } void readSerialPort(){ while (SerialBT.available()) { delay(10); if (SerialBT.available() >0) { char c = SerialBT.read(); //gets one byte from serial buffer msg += c; //makes the string readString } } SerialBT.flush(); }
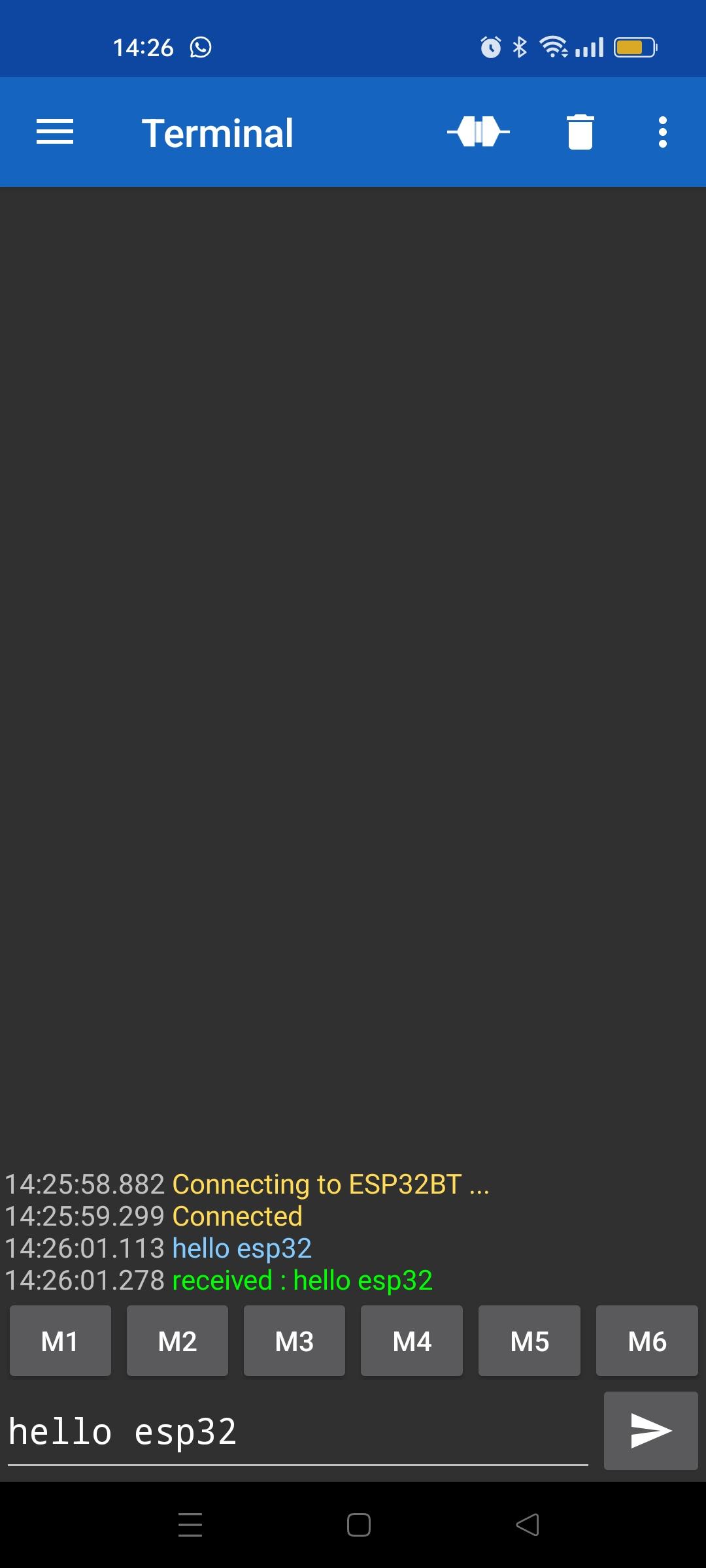
Communicating between ESP32 and Python via Bluetooth
You can manage Bluetooth communication from your PC.
To do this, install the PyBluez package
python -m pip install pybluez
Détecter les appareils Bluetooth
import bluetooth target_name = "ESP32BT" target_address = None nearby_devices = bluetooth.discover_devices(lookup_names=True,lookup_class=True) print(nearby_devices) for btaddr, btname, btclass in nearby_devices: if target_name == btname: target_address = btaddr break if target_address is not None: print("found target {} bluetooth device with address {} ".format(target_name,target_address)) else: print("could not find target bluetooth device nearby")
Output
[('88:C6:26:91:30:84', 'UE\xa0BOOM\xa02', 2360344), ('88:C6:26:7E:F2:7A', 'UE\xa0BOOM\xa02', 2360344), ('4C:EA:AE:D6:92:08', 'OPPO A94 5G', 5898764), ('41:42:DD:1F:45:69', 'MX_light', 2360344), ('3C:61:05:31:5F:12', 'ESP32BT', 7936)] found target ESP32BT bluetooth device with address 3C:61:05:31:5F:12
Connecting and communicating with the ESP32
Here’s a Python script to automatically connect to the ESP32 Bluetooth device from a PC
import bluetooth import socket target_name = "ESP32BT" target_address = None nearby_devices = bluetooth.discover_devices(lookup_names=True,lookup_class=True) print(nearby_devices) for btaddr, btname, btclass in nearby_devices: if target_name == btname: target_address = btaddr break if target_address is not None: print("found target {} bluetooth device with address {} ".format(target_name,target_address)) """ # With PyBluez NOT WORKING serverMACAddress = target_address port = 1 s = bluetooth.BluetoothSocket(bluetooth.RFCOMM) s.connect((serverMACAddress, port)) while 1: text = raw_input() # Note change to the old (Python 2) raw_input if text == "quit": break s.send(text) data = s.recv(1024) if data: print(data) sock.close()""" serverMACAddress = target_address port = 1 s = socket.socket(socket.AF_BLUETOOTH, socket.SOCK_STREAM, socket.BTPROTO_RFCOMM) s.connect((serverMACAddress,port)) print("connected to {}".format(target_name)) while 1: text = input() if text == "quit": break s.send(bytes(text, 'UTF-8')) data = s.recv(1024) if data: print(data) s.close() else: print("could not find target bluetooth device nearby")
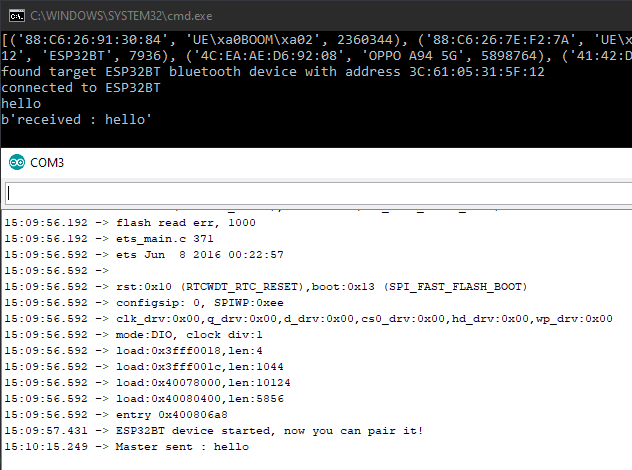
N.B.: Only the socket library works for Bluetooth communication. There seems to be a maintenance issue with the PyBluez library.