,
The C language pointer is a very powerful tool for carrying out certain tasks. It’s a concept you need to grasp if you want to improve your C skills. Some algorithms will be more complicated or even impossible to write without the use of pointers.
Introduction
In a program, any defined element (variables, objects, functions, etc.) is stored in memory at a specific address. To find this element, all you need to know is the memory address used by the element, or the first address used if the variable takes up several memory areas.
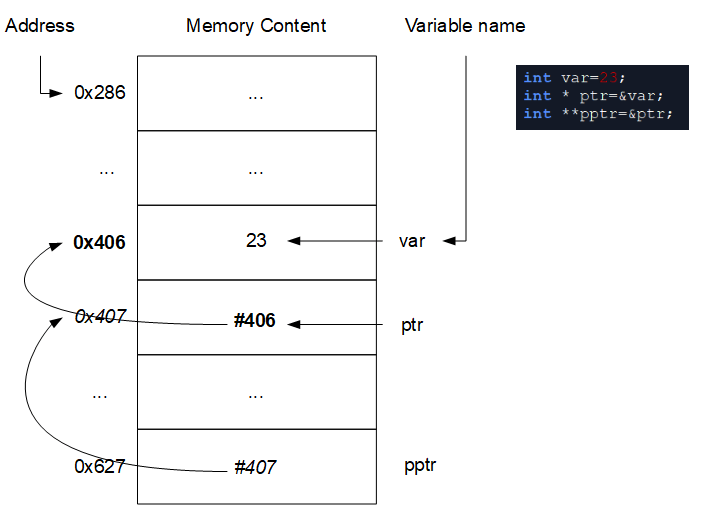
A pointer is a variable containing the memory address of another variable (or object). Although the value of a pointer is always an integer, its type depends on the object to which it points.
Declaring a pointer
The type of the pointer must be identical to the type of the variable it addresses.
<type> * <pointer_name> //or <type> *<pointer_name>
Ex:
float myfloat float * myfloataddress; int myint int * myintaddress;
We can therefore initialize the variable and its address on the same line
int i,*pi;
Operation on pointers
The address of a variable is an integer. It is therefore possible to add and subtract an integer to an address in order to obtain a pointer of the same type to a different address.
ptr+i -> address contained in ptr + i * size of ptr type
You can also subtract two addresses that point to the same type. Subtraction is useful, for example, when the two pointers point to the same array, to find out the number of elements between the two elements pointed to.
ptr1-ptr2 -> (address pointed to by ptr1 – address pointed to by ptr2)/( type size of ptr1 and ptr2)
It is also possible to compare address values using the operators <,>,<=,>=,!= and ==.
Modify the value of a variable using a pointer
In the following example, x is an integer variable, p is an integer pointer containing the address of variable x. The value of x, initialized at 3, is changed to 21 using the pointer.
int x=3; int * p=&amp;x; *p=21; // is equivalent to x=21; printf("valeur de *p = %d\n",*p); // equivalent to printf("valeur de x = %d\n",x);
Modify a function input variable
In C, a function can only return a single variable. To return several values, it is possible to use the pointers of these input variables rather than returning the values themselves.
#include <iostream> int myparam=5; struct mystruct_T{ int val1; int val2; }STRUCT; void change_struct( mystruct_T * mystruct, int * param) { mystruct->val1=10; *param=26; } int main(){ change_struct(&STRUCT, &myparam); printf("Struct.val1 : %d",STRUCT.val1); printf("param : %d",myparam); }
With this method, you can modify several variables as if the function returned several values.
Brief summary
- A pointer is a variable that contains the address of another variable.
- To access the address of the var variable we use the address operator(&): &var
- To get the value of the variable from the address, use the indirection(*) operator: *pointer
- When a structure pointer is used, the -> operator is used to access the structure member: mystruct->elm1
- It is possible to point to functions