One of the main interests of programming languages, like Python, is to automate tasks like sending mail. In this tutorial we will see how to write a Python program to send an email from a Gmail account.
This tutorial can be applied to any computer including a Raspberry Pi.
To send mails from Arduino compatible microcontroller. You can read this tutorial.
Material
- Computer or Raspberry Pi
- Gmail account
Principle of operation
To send a mail with Python, we will use the SMTP (Simple Mail Transfer Protocol), so we will use a server that manages the sending of mail. Like the Gmail smtp server. To use this server, you need a Gmail account (free creation) and you need to activate the two-step validation.
Gmail account configuration
On the account management page, go to the “Security” tab, then look for the “Sign in to Google” box.
Add two-step validation.
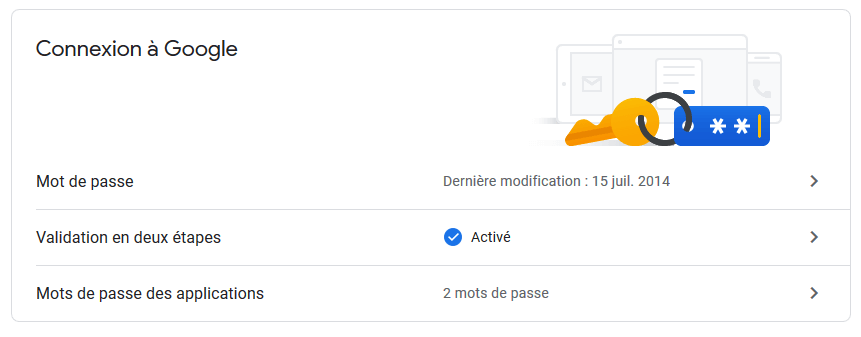
Generate an account access password for a given application
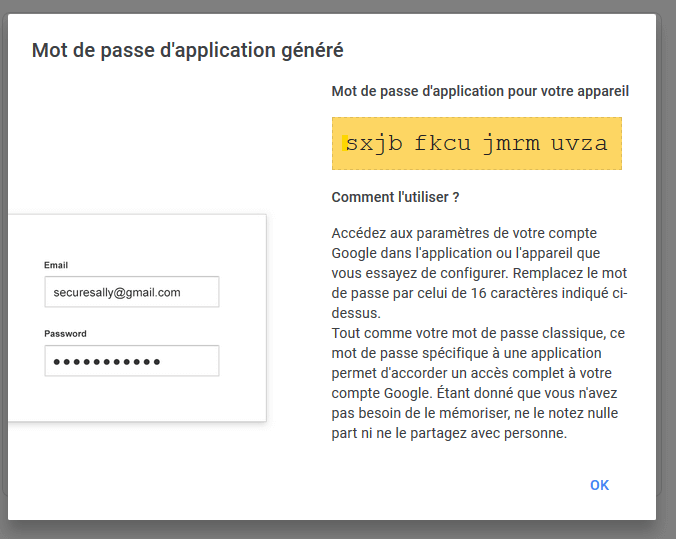
You just have to copy in the Python code below, the user ID (the Gmail address) and the password issued by Google (yellow insert above).
This function is voluntarily simplified to show the sending of mail but it is possible to modify it to send different subject, message body and information.
Code
To manage the STMP protocol, we will use the stmplib library that you can install with the command
pip install smtplib
For a simpler reuse of the code, we have created the EmailSenderClass to facilitate the sending of mail. Don’t forget to replace the recipient’s address “destinataire@email.com” with your own at the bottom of the script in the function
email.sendHtmlEmailTo("Admin","destinataire@email.com","Ceci est un mail automatique envoyé à partir d'un script Python.")
#!/usr/bin/env python # -*- coding: utf-8 -*- #Libraries import sys import smtplib from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText from email.mime.base import MIMEBase from email import encoders from email.header import Header from email.utils import formataddr EMAIL_HTML_TEMPLATE="""<html> <head> </head> <body> <p style ="margin: 5px 0;line-height: 25px;">Bonjour {},<br> <br> Ceci est un mail au format html. <br> {} <br> Bien à vous,<br> {} <br> </p> </body> </html> """ class EmailSenderClass: def __init__(self): """ """ self.logaddr = "<gmail address>" self.fromaddr = "contact@domaine.com"# alias self.password = "<gmail app password"# def sendMessageViaServer(self,toaddr,msg): # Send the message via local SMTP server. server = smtplib.SMTP('smtp.gmail.com', 587) server.starttls() server.login(self.logaddr, self.password) text = msg.as_string() server.sendmail(self.fromaddr, toaddr, text) server.quit() def sendHtmlEmailTo(self,destName,destinationAddress,msgBody): #Message setup msg = MIMEMultipart() msg['From'] = "Me<"+self.fromaddr+">" msg['To'] = destinationAddress msg['Subject'] = "Hello mail" hostname=sys.platform txt = EMAIL_HTML_TEMPLATE txt=txt.format(destName,msgBody,hostname) #Add text to message msg.attach(MIMEText(txt, 'html')) print("Send email from {} to {}".format(self.fromaddr,destinationAddress)) self.sendMessageViaServer(destinationAddress,msg) if __name__ == "__main__": email= EmailSenderClass() email.sendHtmlEmailTo("Admin","destinataire@email.com","Ceci est un mail automatique envoyé à partir d'un script Python.")
Result
After running the script, you should receive in your inbox the email described in the program.
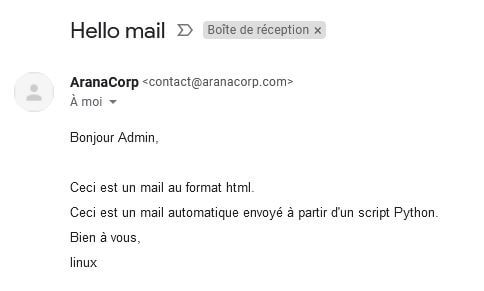