The Arduino FlexiTimer2 library is a library that allows to activate functions at regular time intervals. It allows, as such, to make multitasking programs with Arduino microcontrollers. This method is useful when you want to operate two motors in parallel independently.
Material
- Arduino UNO
- USB A/ USB B cable
Description
The FlexiTimer2 library is based on the MsTimer2 library. It contains a set of functions that allow to interface with the timer2 register of the Arduino’s ATmega microprocessor. This library, similar to a structure with the millis() function, calls a function when the specified time interval has elapsed.
The interest of this library compared to MsTimer2 is that it is possible to modify the counting resolution, i.e. the frequency at which the counter is incremented.
Code
In this code, we will create a void onTimer function, which will allow us to change the state of the Arduino’s embedded LED. To initialize the timer, we use the line:
FlexiTimer2::set(500, onTimer);
And to activate it, the command:
FlexiTimer2::start();
#include <FlexiTimer2.h> unsigned long elapsedTime, previousTime; void onTimer() { static boolean state = HIGH; elapsedTime=millis()-previousTime; Serial.print(F("Set LED 13 : ")); if(state){ Serial.print(F("ON")); }else{ Serial.print(F("OFF")); } Serial.print(F(" - "));Serial.print(elapsedTime);Serial.println(F("ms")); digitalWrite(13, state); state = !state; previousTime=millis(); } void setup() { Serial.begin(9600); pinMode(13, OUTPUT); FlexiTimer2::set(500, onTimer); // equivalent to FlexiTimer2::set(500, 1/1000, onTimer); FlexiTimer2::start(); } void loop() { }
N.B.: We have added an elapsed time measurement for illustration purposes, but care must be taken when using interrupt-based functions in interrupt-call functions. There can be interferences.
Result
Once the program is started, the on-board LED turns on and off every 500ms
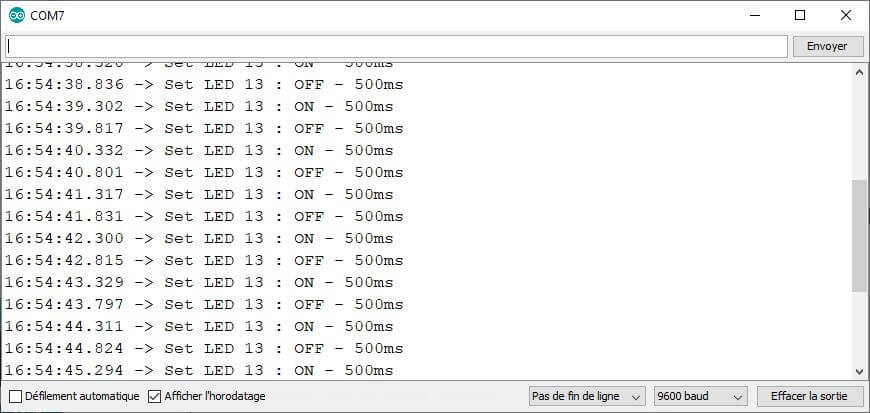
Sources
Retrouvez nos tutoriels et d’autres exemples dans notre générateur automatique de code
La Programmerie