, ,
We have seen that the ESP32 NodeMCU is easily programmable using the Arduino IDE. We will see how to program it with MicroPython. The advantage of using Python to program an ESP32 is to use it to its full potential.
Before following this tutorial, you must first install Python 3.
In this tutorial we will see how to configure the ESP32, how to use a terminal to test Python commands. Finally, we will see two methods to load and run Python scripts on the microcontroller.
Presentation of MicroPython
MicroPython is an implementation of Python 3 created to work in the microcontroller environment and its constraints. It has a subset of the Python libraries as well as libraries to interface with low level hardware and network interfaces. (ex: Wifi)
Cards supporting MicroPython:
- ESP8266 Boards (ex: NodeMCU ESP8266)
- PyBoard
- Micro:Bit
- Teensy 3.X
- WiPy – Pycom
- Raspberry Pi Pico
Install MicroPython with Thonny IDE
The easiest way to install and use MicroPython on ESP32 (and other microcontrollers) is to use the Thonny IDE software. Indeed you can install the firmware from the software interface and use it to develop your code and upload it to the board.
Download the firmware corresponding to your microcontroller.
To install the firmware, go to the “Run” menu and select “Select interpreter…”
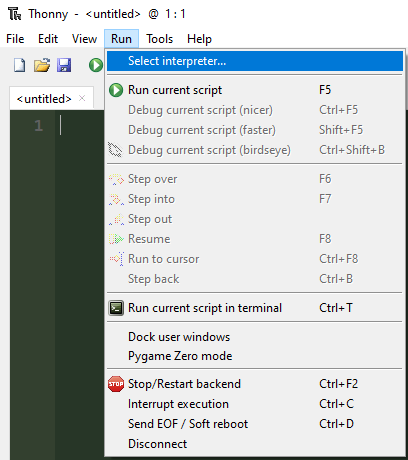
Then press “Install or update firmware” at the bottom right
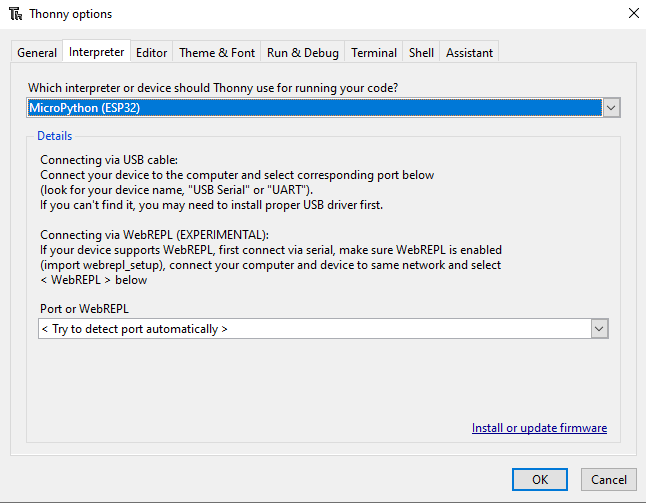
Select your card and the file containing the previously downloaded firmware. Then press “Install”.
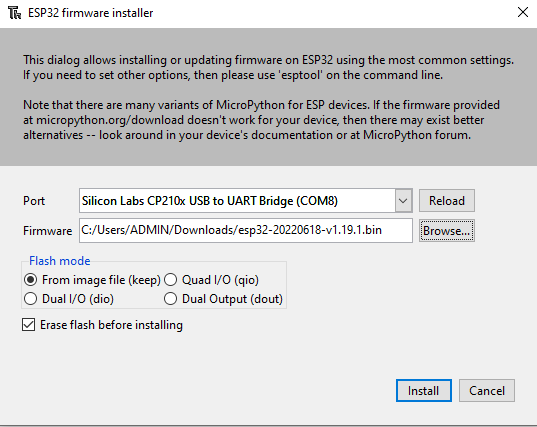
Once the firmware is installed, you can enter Python commands in the console.
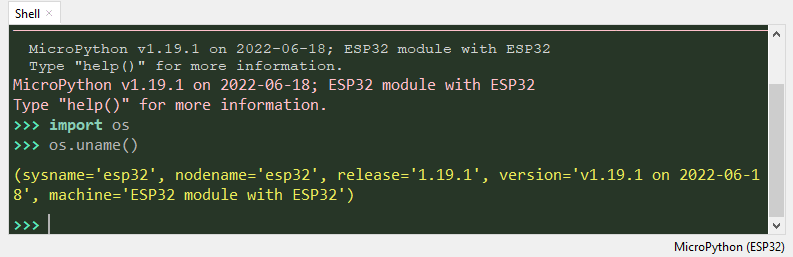
You can also write your script and run it on the map from Thonny. Copy the following code into the editor
import sys import time def main(): print("Program is running ") time.sleep(0.5) if __name__=="__main__": print("{} initialized".format(sys.platform)) while(1): try: main() except KeyboardInterrupt: print("Program stopped") sys.exit(0)
To run the script, go to “Run>Run current script” or F5. Rename the script main.py
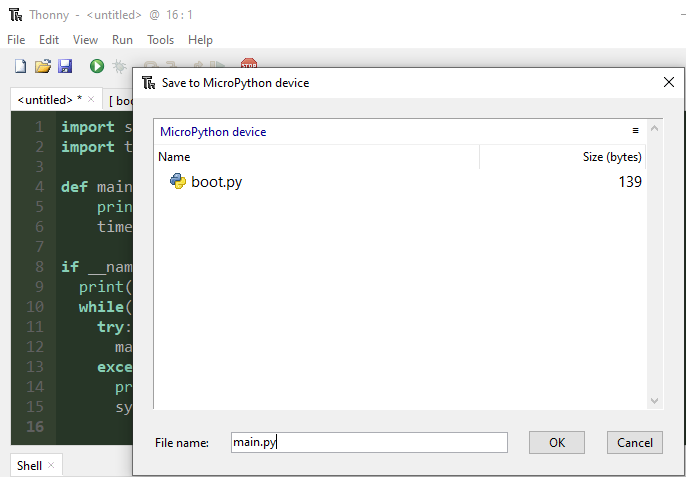
The code should run automatically
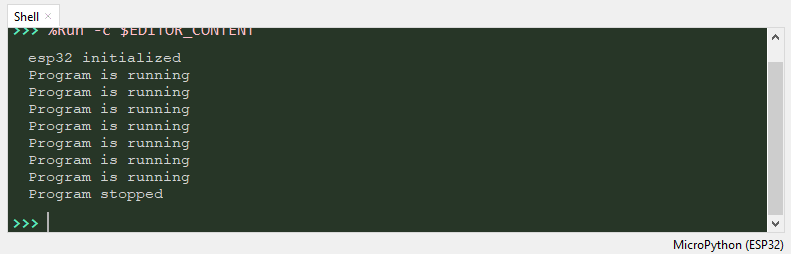
When you restart the IDE, you can open the files on the card that you can find with the command
import os
os.listdir()
N.B.: To be able to program the microcontroller with Arduino IDE, you must upload an Arduino code. This will reload the bootloader in the flash.
Install the firmware on the ESP32 by command lines
To use MicroPython, we will erase the flash memory of the ESP32 and install the firmware. To do this, we will use the esptool tool (.py or .exe depending on what you want to use)
- Install esptool.py
python3 -m pip install esptool
or esptool.exe (available when installing the esp package on Arduino IDE)
- Erase flash memory (Warning: the port name may be different from yours)
python3 -m esptool --chip esp32 --port COM9 erase_flash
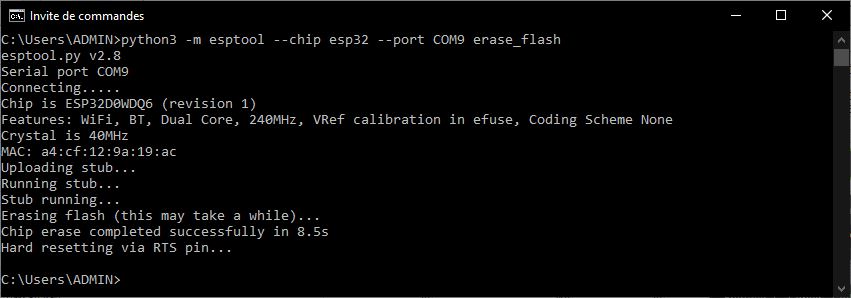
- Download the firmware, then enter the following line of code (Warning: the port name and file address may be different from yours)
python3 -m esptool --chip esp32 --port COM9 --baud 460800 write_flash -z 0x1000 C:\Users\ADMIN\Downloads\esp32-idf3-20210202-v1.14.bin
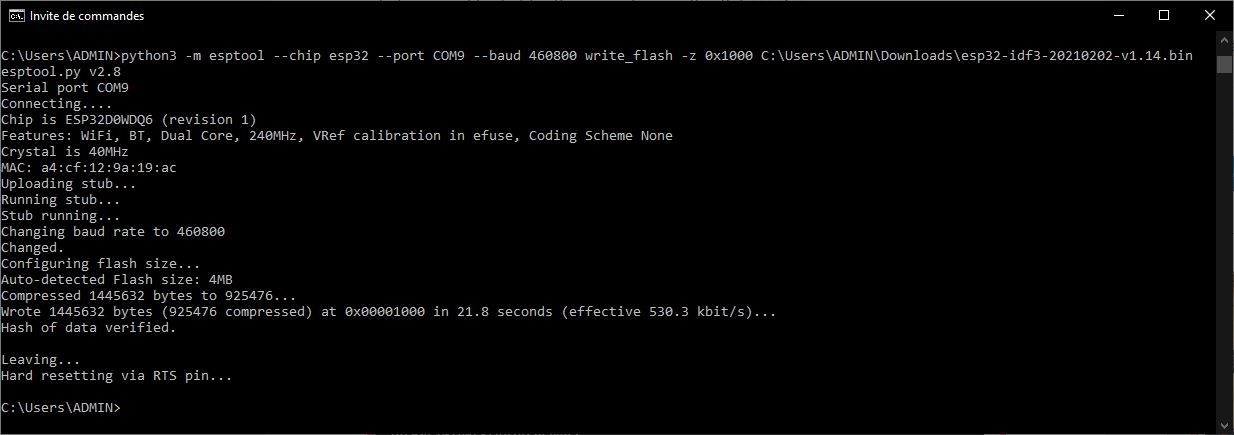
To be able to communicate and program the ESP with MicroPython, we need to install a terminal or an IDE. We will see here the use of PuTTy and TeraTerm.
Use of different tools to communicate and program your ESP32
Once your microcontroller is configured with Micropyhton, you can use different tools to program in Python. Terminals like TeraTerm or Putty and IDEs like VS Code, uPyCraft or Thonny.
Install the TeraTerm Terminal on Windows
Download and install TeraTerm
Open TeraTerm then select the serial port corresponding to the microcontroller.
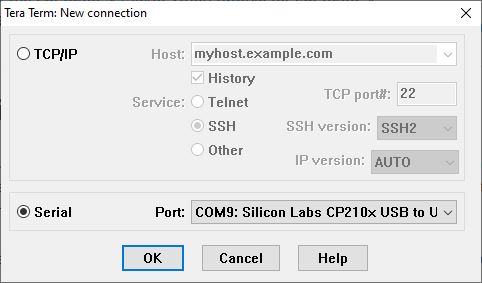
Configure, then, the serial communication dans Setup> Serial Port …
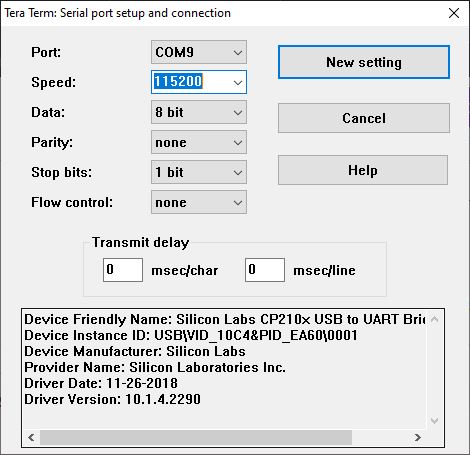
You can now use the terminal to enter Python commands and execute them on the ESP32
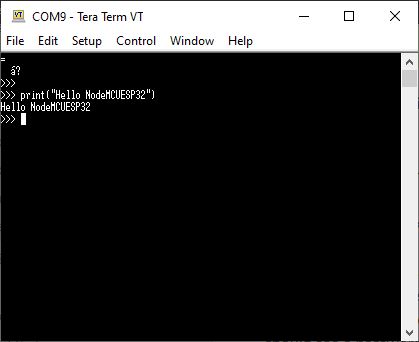
Installation of the PuTTy terminal
Download and install PuTTy
Open Putty and select the serial port corresponding to the microcontroller.
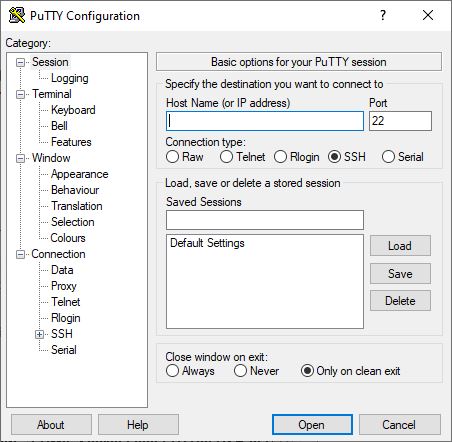
Configure, then, the serial communication
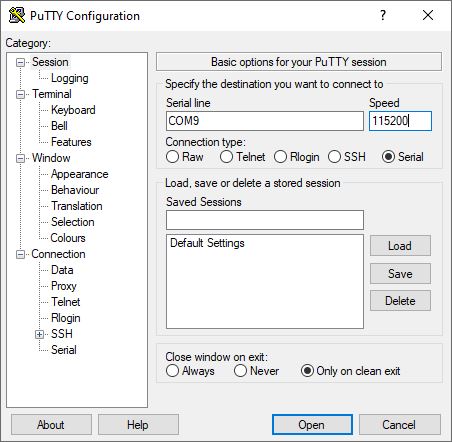
You can now use the terminal to enter Python commands and execute them on the ESP32
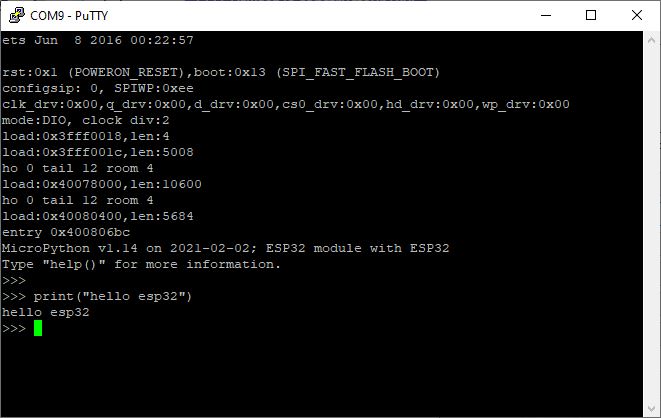
The PuTTy and TeraTerm terminals are two good options for testing a few Python commands but not for making a complete program.
Create a Python script and upload it with ampy
We are going to write a simple code in order to test the uploading of a code on ESP32. There are two files that are treated differently by ESP32: boot.py and main.py. By default, only boot.py is present. The boot.py runs first and then the main.py is executed in turn. If you want to create a script that runs at startup you must call it main or run it in main. If you don’t want it to run at startup call it something else.
import sys import time def main(): print("Program is running ") time.sleep(0.5) if __name__=="__main__": print("{} initialized".format(sys.platform)) while(1): try: main() except KeyboardInterrupt: print("Program stopped") sys.exit(0)
We will then install ampy which will allow us to load a file on the microcontroller.
python3 -m pip install adafruit-ampy
Once ampy is installed, you can use it in a command prompt with the “put” function to load a file by specifying its path and name.
ampy -d 0.5 --port COM9 put \main.py

N.B.: If you encounter the error “ampy.pyboard.PyboardError: could not enter raw repl”, you can increase the delay to 1 or 2 (i.e. “-d 1” or “-d 2”)
If you connect to the ESP32 with a terminal, you will see the program running (you can stop the script with Ctrl+C)
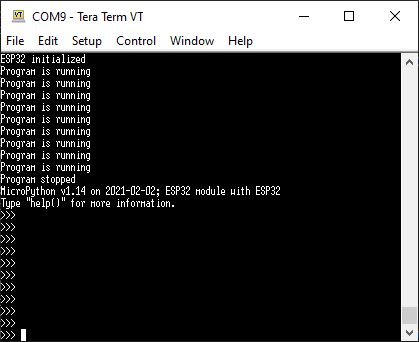
The main.py script will run on each reboot. To remove it, enter the following command in the terminal
import os
os.remove('main.py')
Using the uPyCraft IDE
When developing code and it is necessary to test it, it is complicated to go through the command prompt to load it each time. There is an IDE for Python, similar to the Arduino IDE: uPyCraft that you can use as an editor and as a terminal to interface with the microcontroller.
- Download and open uPyCraft
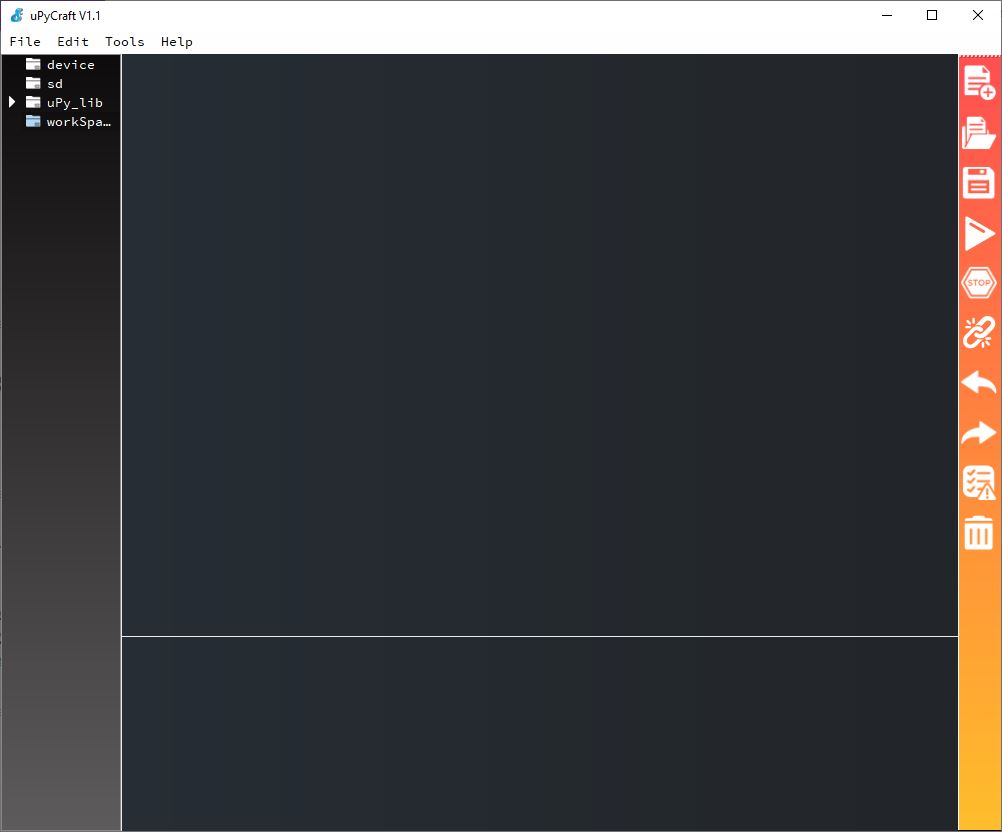
- Dans Tools> Preferences, vérifiez la configuration du port série
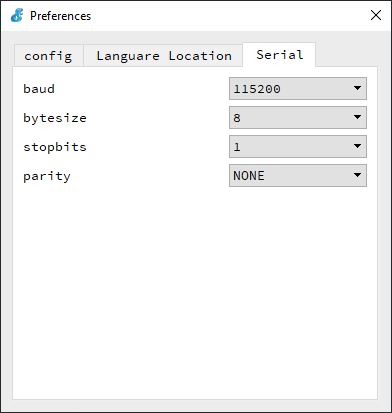
- Dans Tools>Serial, sélectionnez le port série utilisé par le microcontrôleur
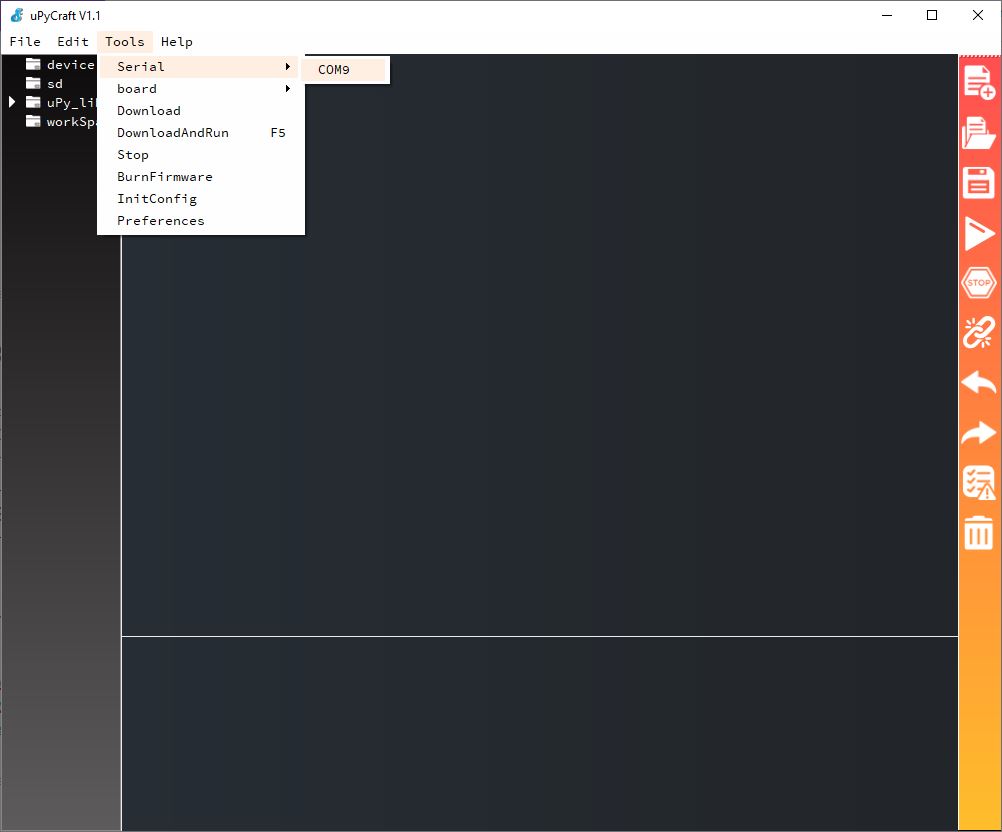
Once the microcontroller is connected to the interface, you can use the console as a terminal
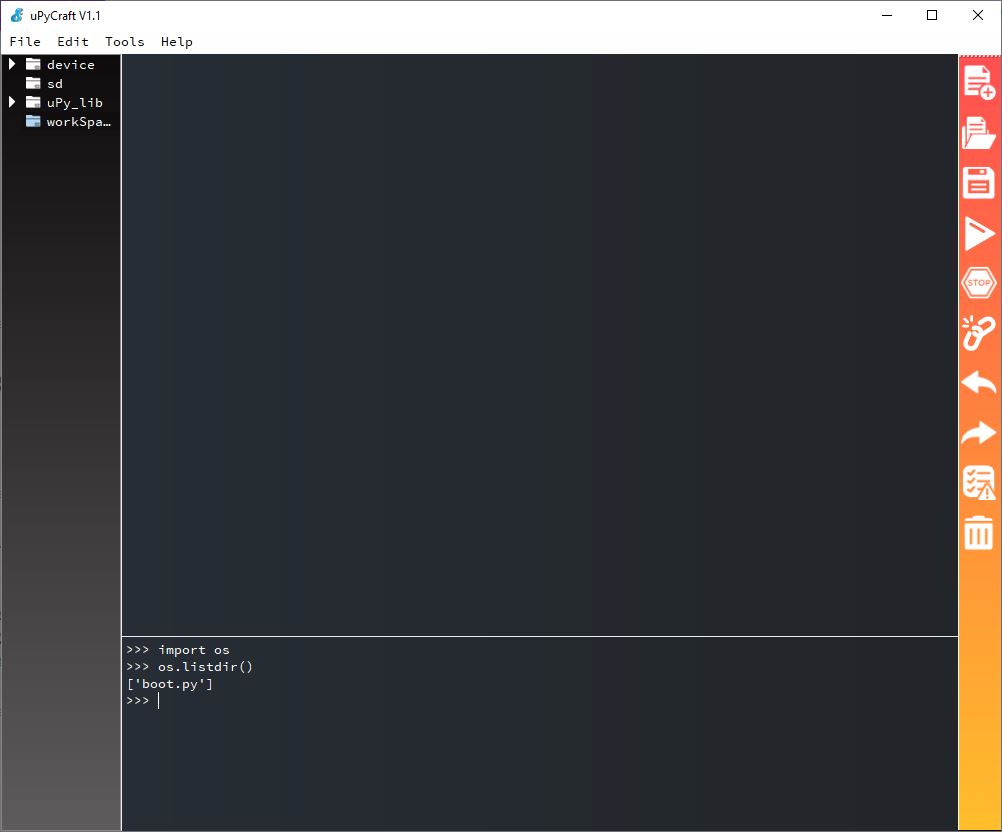
You can also create and edit a file containing the Python script. Once the code is written, you can load it and execute it by pressing F5. To validate the code upload, it may be necessary to press the reset button on the board. You can stop the code by pressing Ctrl+C in the console.
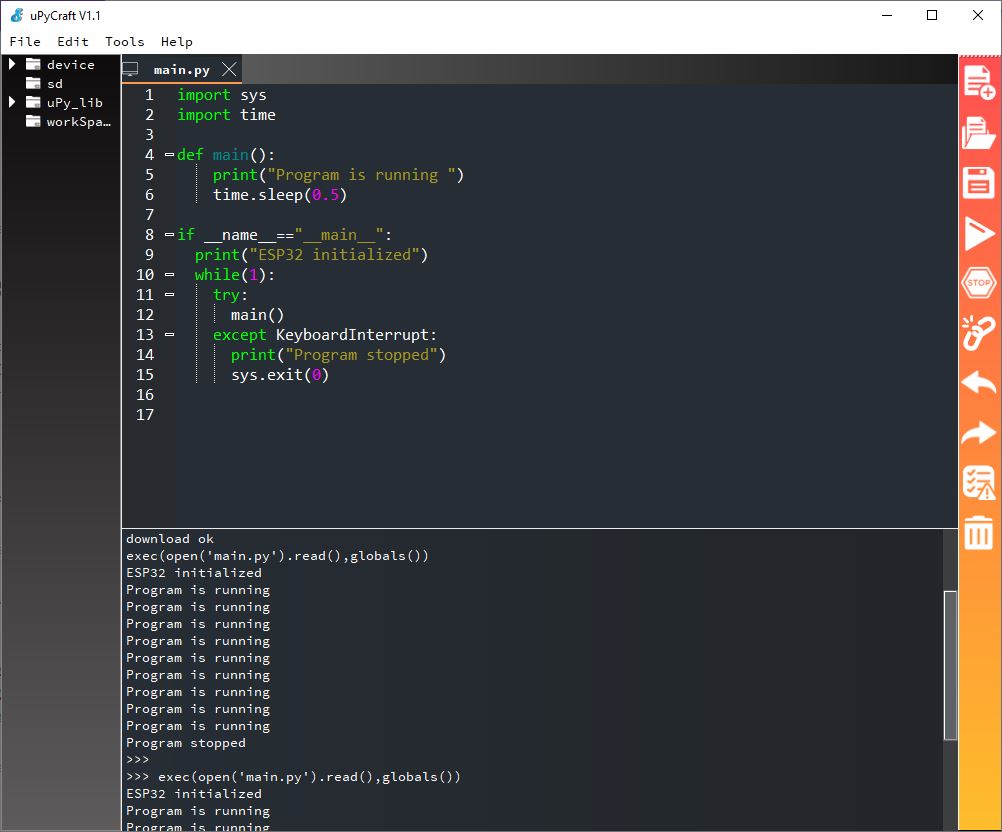
N.B.: You can restart the script without doing a reset, by entering the command
exec(open('main.py').read(), globals())
Delete the file
If you don’t want the script to be triggered on restart, you have to delete the main.py code by using the command in a terminal or in the IDE
import os
os.remove('main.py')
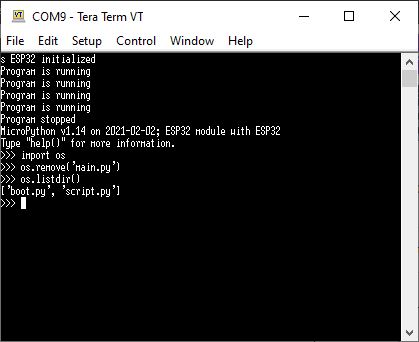
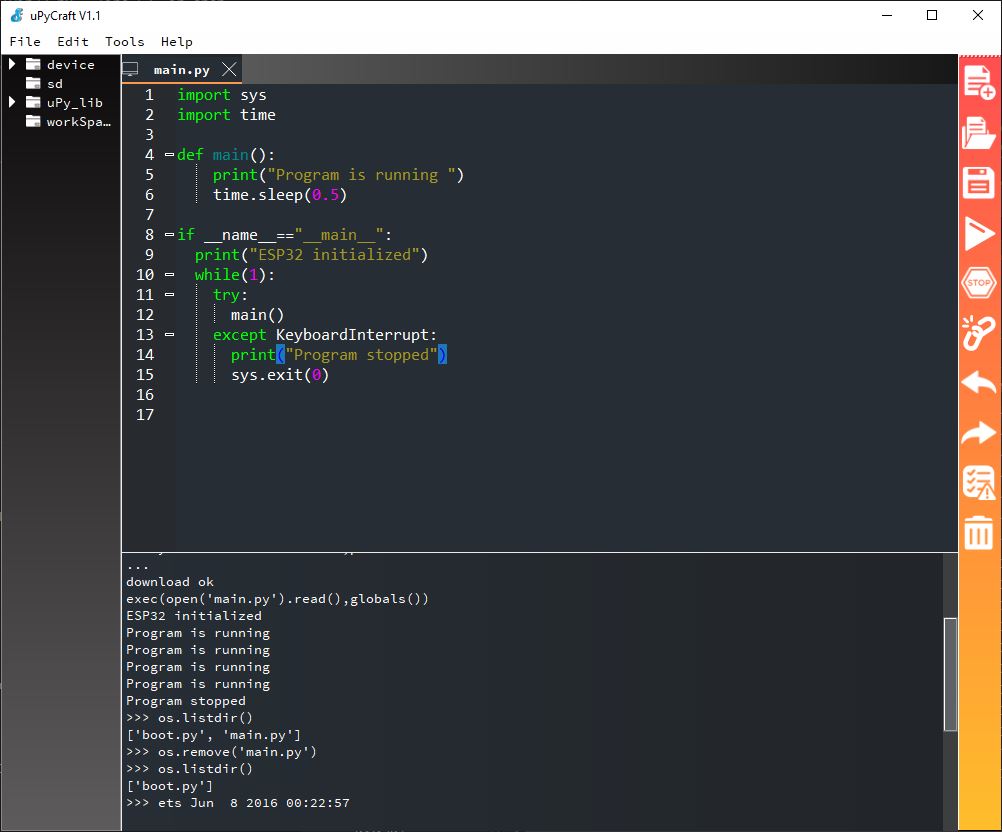
Reset to default configuration
To be able to program the microcontroller with Arduino IDE, you must delete the main.py file if it exists and upload an Arduino code. This will reload the bootloader in the flash.
To use MicroPython again, the flash memory must be erased and the firmware loaded.