The if statement is the basic conditional structure and is found in all programming languages. It allows you to execute different blocks of code according to a condition. It is the basis of many algorithms and allows a computer to make a choice based on the data sent to it.
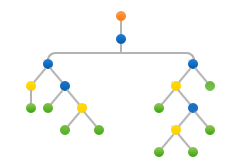
Syntax of the if statement
The if statement takes, as input, a condition in the form of a boolean variable and executes a block of instructions if it is true (in this case we say that the condition is fulfilled) and another if it is false.
if(condition){ <liste d'instructions si condition=true>; }else{ <liste d'instructions si condition=false>; }
Example of a code determining the sign of a variable with the if statement:
int val=-10; void setup() { Serial.begin(9600); } void loop() { if(val>=0){ Serial.println(F("Value is positive")); }else{ Serial.println(F("Value is negative")); } val=val+2; //increment val if(val>10) val=-10; // set val to initial value delay(500); //delay for monitor display }
A boolean is a type of variable equal to true( 1-true) or false(0-false). In the C(Arduino) language, boolean variables are initialized using the keyword bool or boolean. A boolean can be obtained in different ways
- The result of a logic test <,>,==,!=,!
condition = variable == 10; // variable égale à 10? condition = temperature>30; // temperature est supérieure à 30 condition = vitesse <= 100 // vitesse inférieure ou égale à 100 condition = !true // condition égale non vraie (faux)
- The result of a function
bool test(int val){ //retourne vraie si la valeur est supérieur à 200 return val>200; }
- The result of several conditions
condition=(temperature>30) && (vitesse<20);
Other syntaxes
The if statement can have several syntaxes depending on the case.
It is possible to forget the braces when the only instruction is on one line.
if(condition) <instructions>;
Example:
if(condtion) Serial.println("Conditon est réalisée");
If there is no instruction to execute when the condition is not fulfilled, it is possible to forget the else{} block.
if(condition){ <liste d'instructions>; }
If several conditions are evaluated at the same time
if(condition){ // <liste d'instructions si condition==true>; }else if(condition2){ //<liste d'instructions si condition==false && condition2==true>; }else if(condition3){ //<liste d'instructions si condition==false && condition2==false && condition3==true>; }else{ //<liste d'instructions si aucune condition réalisée>; }
Things to remember
- the if statement takes as input a condition represented by a boolean variable
- The condition can be a function that returns a boolean or the result of a test with the logical operators =, !=, <, >, !
- the condition is between brackets
- the instruction block is between braces {}
- If the instruction list is on a single line then there is no need for braces
Bonus
In simple cases, it is possible to use the “?” statement with the following syntax
resultat = (condition) ? <instruction si vraie> : <instruction si faux>
Example, line of code that returns the sign of a variable:
int signe= (variable>=0) ? 1:-1; // retourne 1 si la valeur est positive et - 1 si elle est négative
Make sure you understand booleans and logical operators to use the if statement correctly. Once you have mastered this statement, your algorithms will be able to make good decisions.