The sensor DHT11 can measure temperature and humidty in a room. Here is a tutorial on how to retrive the sensor measurement with a Arduino board.
Material
- Computer
- Arduino UNO
- USB cable
- sensor DHT11
- Jumper cables male/male
- breadboard
Wiring DHT11
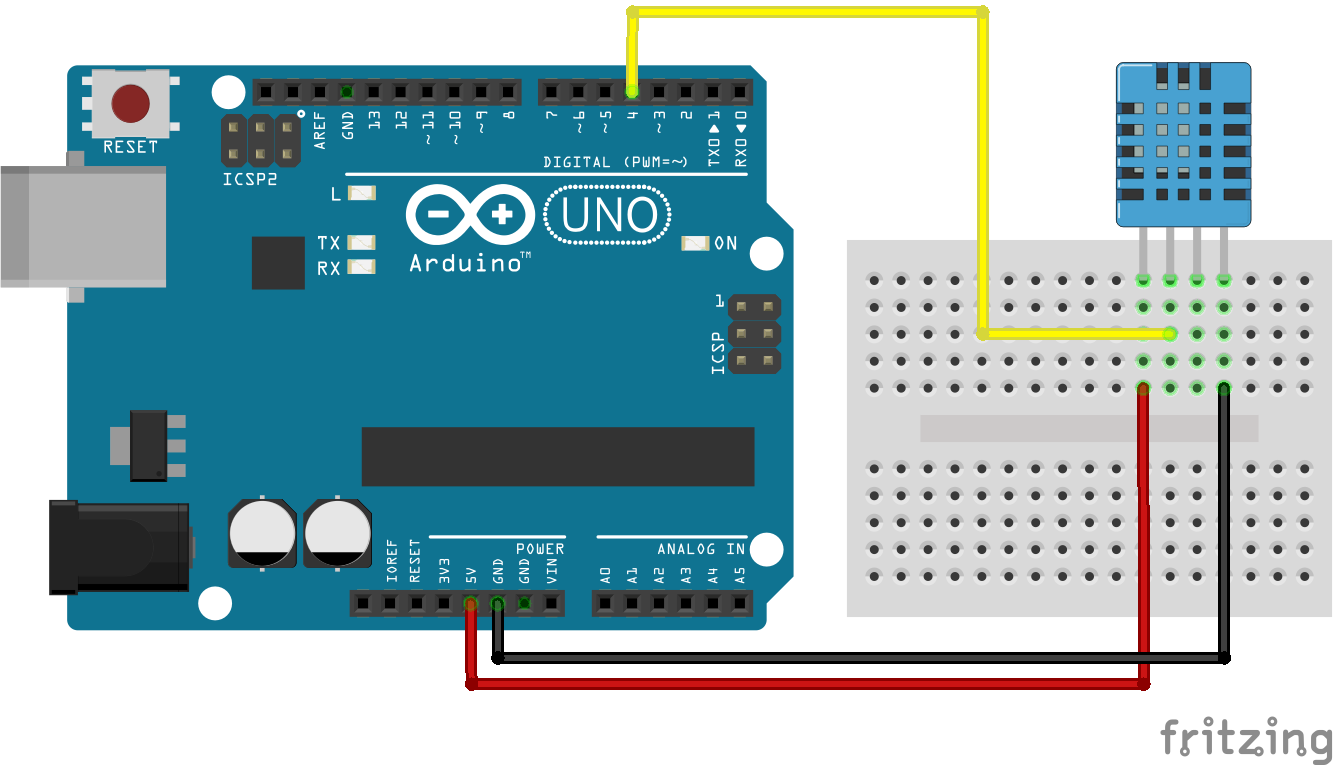
Code
The program to read the measurement of the sensor DHT11 is quite complex and require a bit of work. In such case, the easiest way is to use a library developped by Arduino or other users. Those libraries can come from many sources: Arduino, of course, but also GitHub which is a reference in code exchange. In our case, we copied code from Arduino.
Create library folder
Libraries are often available as downlodable ZIP files that need to be exctrated in the IDE library folder (usually .\Documents\Arduino\libraries). To use the raw code cpied from a random source, we need to manually create the .h and .cpp files, in a folder DHT11lib, in which we will copy-paste the code from the website.

dht11.h
// // FILE: dht11.h // VERSION: 0.4.1 // PURPOSE: DHT11 Temperature & Humidity Sensor library for Arduino // LICENSE: GPL v3 (https://www.gnu.org/licenses/gpl.html) // // DATASHEET: https://www.micro4you.com/files/sensor/DHT11.pdf // // URL: https://playground.arduino.cc/Main/DHT11Lib // // HISTORY: // George Hadjikyriacou - Original version // see dht.cpp file // #ifndef dht11_h #define dht11_h #if defined(ARDUINO) && (ARDUINO >= 100) #include <Arduino.h> #else #include <WProgram.h> #endif #define DHT11LIB_VERSION "0.4.1" #define DHTLIB_OK 0 #define DHTLIB_ERROR_CHECKSUM -1 #define DHTLIB_ERROR_TIMEOUT -2 class dht11 { public: int read(int pin); int humidity; int temperature; }; #endif // // END OF FILE //
dht11.cpp
// // FILE: dht11.cpp // VERSION: 0.4.1 // PURPOSE: DHT11 Temperature & Humidity Sensor library for Arduino // LICENSE: GPL v3 (https://www.gnu.org/licenses/gpl.html) // // DATASHEET: https://www.micro4you.com/files/sensor/DHT11.pdf // // HISTORY: // George Hadjikyriacou - Original version (??) // Mod by SimKard - Version 0.2 (24/11/2010) // Mod by Rob Tillaart - Version 0.3 (28/03/2011) // + added comments // + removed all non DHT11 specific code // + added references // Mod by Rob Tillaart - Version 0.4 (17/03/2012) // + added 1.0 support // Mod by Rob Tillaart - Version 0.4.1 (19/05/2012) // + added error codes // #include "dht11.h" // Return values: // DHTLIB_OK // DHTLIB_ERROR_CHECKSUM // DHTLIB_ERROR_TIMEOUT int dht11::read(int pin) { // BUFFER TO RECEIVE uint8_t bits[5]; uint8_t cnt = 7; uint8_t idx = 0; // EMPTY BUFFER for (int i=0; i< 5; i++) bits[i] = 0; // REQUEST SAMPLE pinMode(pin, OUTPUT); digitalWrite(pin, LOW); delay(18); digitalWrite(pin, HIGH); delayMicroseconds(40); pinMode(pin, INPUT); // ACKNOWLEDGE or TIMEOUT unsigned int loopCnt = 10000; while(digitalRead(pin) == LOW) if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; loopCnt = 10000; while(digitalRead(pin) == HIGH) if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; // READ OUTPUT - 40 BITS => 5 BYTES or TIMEOUT for (int i=0; i<40; i++) { loopCnt = 10000; while(digitalRead(pin) == LOW) if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; unsigned long t = micros(); loopCnt = 10000; while(digitalRead(pin) == HIGH) if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; if ((micros() - t) > 40) bits[idx] |= (1 << cnt); if (cnt == 0) // next byte? { cnt = 7; // restart at MSB idx++; // next byte! } else cnt--; } // WRITE TO RIGHT VARS // as bits[1] and bits[3] are always zero they are omitted in formulas. humidity = bits[0]; temperature = bits[2]; uint8_t sum = bits[0] + bits[2]; if (bits[4] != sum) return DHTLIB_ERROR_CHECKSUM; return DHTLIB_OK; } // // END OF FILE //
Usage of the library
Once the library created and present in the IDE, we can create the .ino file in which we use the library. We slightly adapt the example from the websiteto fit our case.
#include <dht11.h> dht11 DHT11; #define DHT11PIN 4 void setup() { Serial.begin(9600); Serial.println("DHT11 TEST PROGRAM "); Serial.print("LIBRARY VERSION: "); Serial.println(DHT11LIB_VERSION); Serial.println(); } void loop() { Serial.println("\n"); int chk = DHT11.read(DHT11PIN); Serial.print("Read sensor: "); switch (chk) { case DHTLIB_OK: Serial.println("OK"); Serial.print("Humidity (%): "); Serial.println((float)DHT11.humidity, 2); Serial.print("Temperature (°C): "); Serial.println((float)DHT11.temperature, 2); Serial.print("Temperature (°F): "); Serial.println(Fahrenheit(DHT11.temperature), 2); Serial.print("Temperature (°K): "); Serial.println(Kelvin(DHT11.temperature), 2); Serial.print("Dew PointFast (°C): "); Serial.println(dewPointFast(DHT11.temperature, DHT11.humidity)); break; case DHTLIB_ERROR_CHECKSUM: Serial.println("Checksum error"); break; case DHTLIB_ERROR_TIMEOUT: Serial.println("Time out error"); break; default: Serial.println("Unknown error"); break; } delay(2000); } //Celsius to Fahrenheit conversion double Fahrenheit(double celsius) { return 1.8 * celsius + 32; } //Celsius to Kelvin conversion double Kelvin(double celsius) { return celsius + 273.15; } // dewPoint function NOAA // reference (1) : http://wahiduddin.net/calc/density_algorithms.htm // reference (2) : http://www.colorado.edu/geography/weather_station/Geog_site/about.htm double dewPointFast(double celsius, double humidity) { double a = 17.271; double b = 237.7; double temp = (a * celsius) / (b + celsius) + log(humidity*0.01); double Td = (b * temp) / (a - temp); return Td; }
N.B.: When copying code from a website, always check if your componant version and wiring are the same to avoid compatibility issues.
N.B.: Other version of DHT11 exists. If you have one of those other versions check the library DHTLib.
Application
- Create a meteo station with a DHT11 sensor and a LCD screen
Sources
Find other examples and tutorials in our Automatic code generator
Code Architect