There are force transducers that are quite simple to use and compatible with microcontrollers such as Arduino. They consist of a load cell and a conversion module. The load cell is a strain gauge sensor that measures force in one direction in space. It is usually accompanied by an HX711 module capable of amplifying the measurement of forces applied to the force cell.
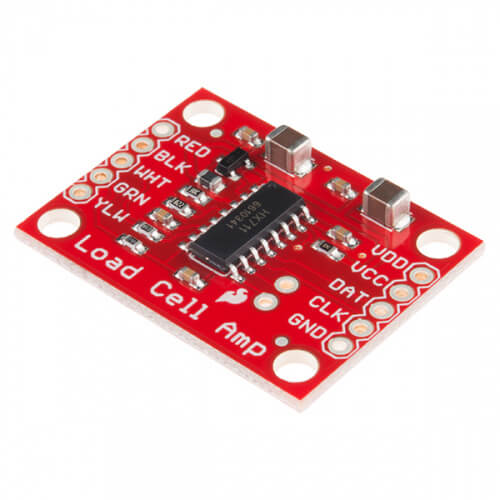
Material
- Computer
- Arduino UNO
- USB cable A Male/B Male
- HX711 module
- Load cell
Principle of operation
The HX711 module is composed of an amplifier and an analog-to-digital converter HX711. It allows the amplification of a signal sent by a load cell. The force sensor uses a Weahstone bridge to convert the force applied to it into an analog signal.
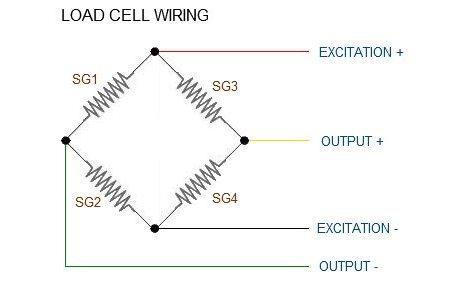
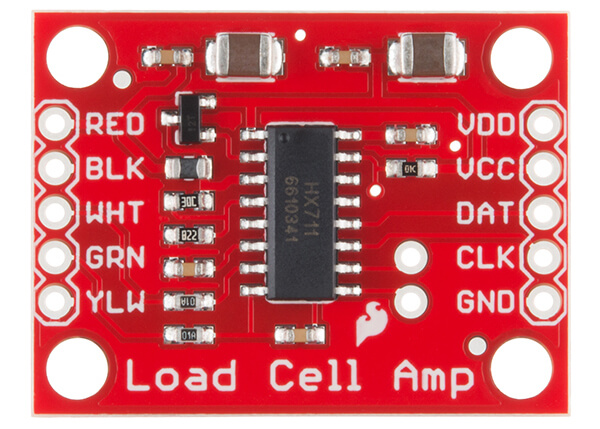
Scheme
The HX711 module communicates with the microcontroller using two digital pins (SCK and DOUT) and is powered by 5V. It is connected to the force cell by 4 wires whose color remains standard on Wheastone bridges:
- Excitation+ (E+) ou VCC RED
- Excitation- (E-) ou GND BLACK ou YELLOW
- Output+ (O+), Signal+ (S+), or Amplificator+ (A+) WHITE
- O-, S-, ou A- GREEN or BLUE
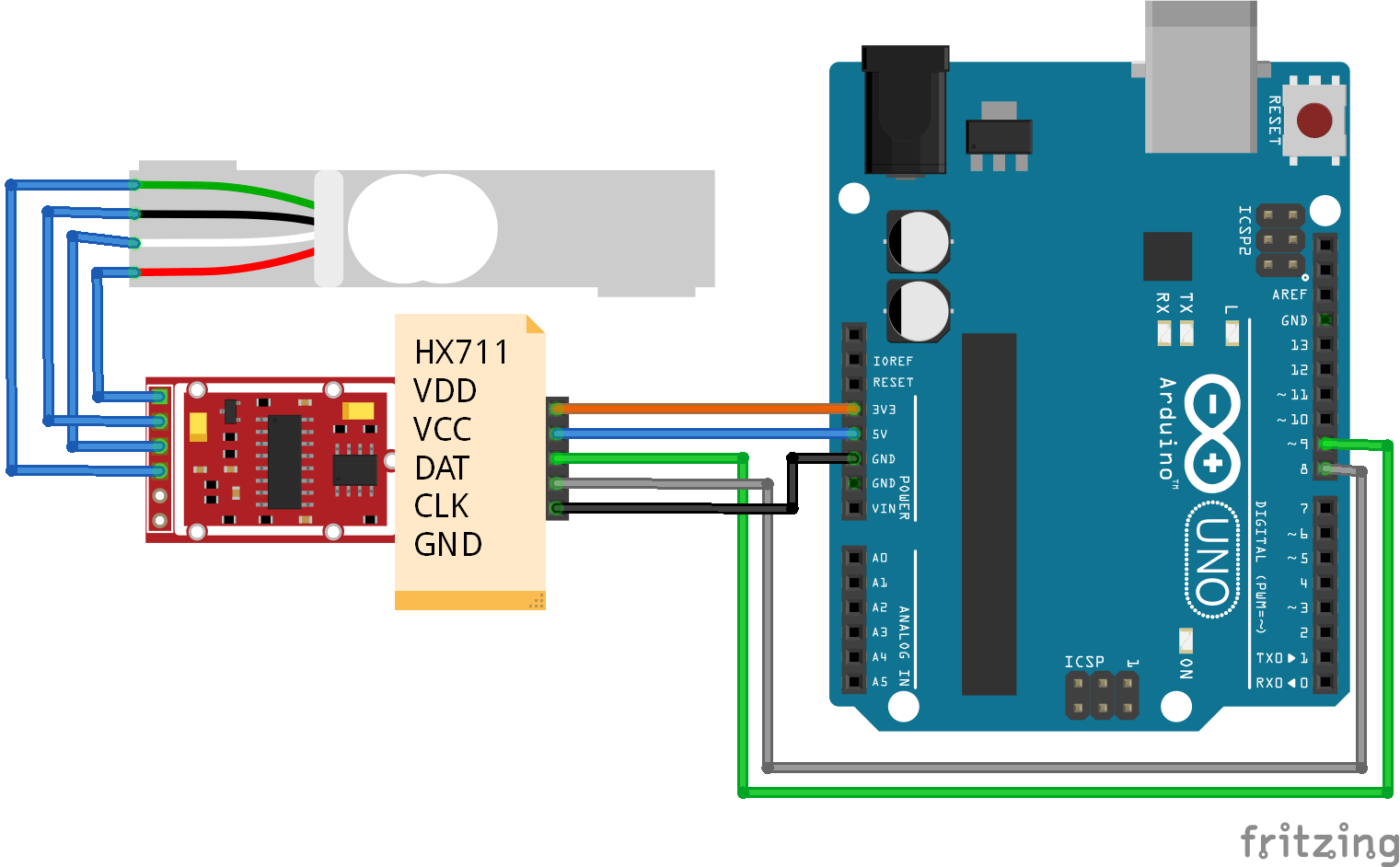
Code
To get a force value from the sensor, it must be calibrated. To perform the calibration, you will have to launch the code with two different known masses in order to calculate the gain and offset of the sensor.
//Libraries #include <HX711.h>//https://github.com/bogde/HX711 //Parameters const long LOADCELL_OFFSET = 340884; const float LOADCELL_DIVIDER = 262.5F; const int numberOfReadings = 10; const int doutPin = 2; const int sckPin = 4; //Variables HX711 scale = ; float weight = 0; float initWeight = 0; void setup() { //Init Serial USB Serial.begin(9600); Serial.println(F("Initialize System")); scale.begin(doutPin, sckPin); scale.set_scale(); //remove scale divider scale.tare(); // reset the scale to 0 //find Serial.println("Put a known wait and send command"); while (Serial.available() == 0) {} Serial.print("Compute average on 10..."); Serial.println(scale.get_units(10), 1); //repeat with two different weight to find DIVIDER and OFFSET //WEIGHT= (val-OFFSET)/DIVIDER scale.set_scale(LOADCELL_DIVIDER); scale.tare(); //scale.set_offset(LOADCELL_OFFSET); delay(200); initWeight = scale.get_units(numberOfReadings * 10), 10; } void loop() { readScale(); } void readScale() { /* function readScale */ //// Read button states from keypad if (scale.is_ready()) { weight = scale.get_units(numberOfReadings), 10; Serial.print("weight : "); Serial.println(weight); } else { Serial.println("HX711 not found."); } }
Result
When the sensor is calibrated, it returns the value in g or kg (or even N) depending on the unit you chose when calculating the sensor parameters. It is possible to smooth the measurement over several values by placing the number of readings desired in the get_units(numberOfReadings) function. This allows to have a more stable and accurate measurement of the effort.
Applications
- Create a connected scale
- Activate a mechanism according to the weight of an object
Sources
Retrouvez nos tutoriels et d’autres exemples dans notre générateur automatique de code
La Programmerie